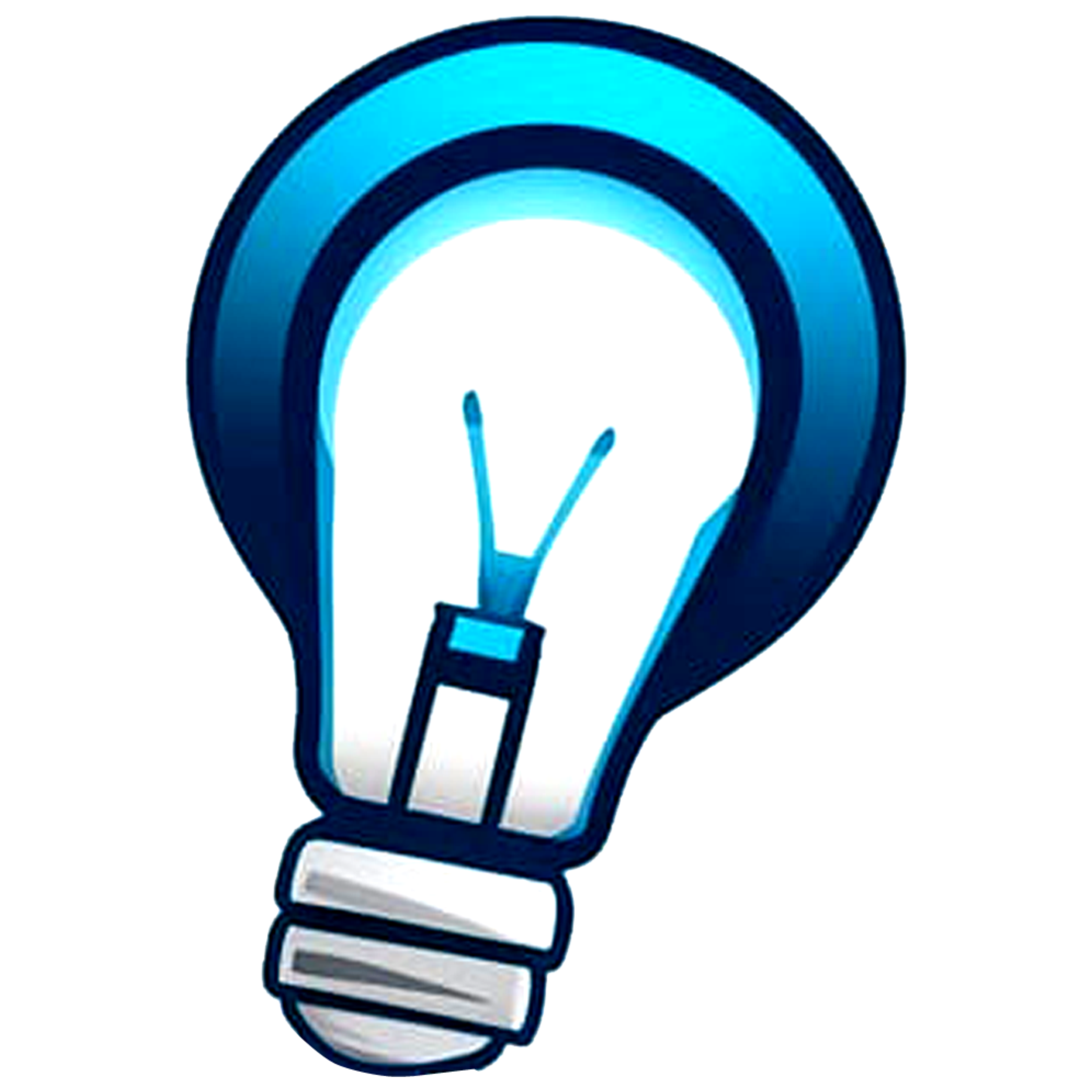
- Home
-
HTML
HTML Introduction HTML Tags HTML Elements HTML Attributes HTML Heading HTML Paragraph HTML Formatting HTML Quotations HTML Comments HTML Styles HTML Color HTML CSS HTML Images HTML Favicon HTML Links HTML DIV HTML Tables HTML Table Size HTML Table Head Table Padding & Spacing Table colspan rowspsn HTML Table Styling HTML Colgroup HTML List HTML Block & Inline HTML Classes HTML Id HTML Iframes HTML Head HTML Layout HTML Semantic Elements HTML Style Guide HTML Forms HTML Form Attribute HTML Form Element HTML input type HTML Computer code HTML Entity HTML Symbol HTML Emojis HTML Charset HTML Input Form Attribute HTML URL Encoding
-
CSS
CSS Introduction CSS Syntax CSS Selector How To Add CSS CSS Comments CSS Colors CSS Background color CSS background-image CSS Borders CSS Margins CSS Height, Width and Max-width CSS Box Model CSS Outline CSS Text CSS Fonts CSS Icon CSS Links CSS Tables CSS Display CSS Maximum Width CSS Position z-index Property
- JavaScript
-
JQuery
What is jQuery? Benefits of using jQuery Include jQuery Selectors. Methods. The $ symbol and shorthand. Selecting elements Getting and setting content Adding and removing elements Modifying CSS and classes Binding and Unbinding events Common events: click, hover, focus, blur, etc Event delegation Using .on() for dynamic content Showing and hiding elements Fading elements in and out Sliding elements up and down .animate() Understanding AJAX .ajax() .load(), .get(), .post() Handling responses and errors. Parent Chlid Siblings Filtering Elements Using find Selecting form elements Getting form values Setting form values Form validation Handling form submissions jQuery plugins Sliders plugins $.each() $.trim() $.extend() Data attributes Debugging jQuery code
-
Bootstrap 4
What is Bootstrap Benefits of using Setting up Container Row and Column Grid Classes Breakpoints Offsetting Columns Column Ordering Basic Typography Text Alignment Text colors Backgrounds Display Font Size Utilities Buttons Navs and Navbar Forms Cards Alerts Badges Progress Bars Margin Padding Sizing Flexbox Dropdowns Modals Tooltips Popovers Collapse Carousel Images Tables Jumbotron Media Object
- Git
-
PHP
PHP Introduction PHP Installation PHP Syntax PHP Comments PHP Variable PHP Echo PHP Data Types PHP Strings PHP Constant PHP Maths PHP Number PHP Operators PHP if else & if else if PHP Switch PHP Loops PHP Functions PHP Array PHP OOps PHP Class & Object PHP Constructor PHP Destructor PHP Access Modfiers PHP Inheritance PHP Final Keyword PHP Class Constant PHP Abstract Class PHP Superglobals PHP Regular Expression PHP Interfaces PHP Static Method PHP Static Properties PHP Namespace PHP Iterable PHP Form Introduction PHP Form Validation PHP Complete Form PHP Date and Time PHP Include Files PHP - Files & I/O File Upload PHP Cookies PHP SESSION PHP Filters PHP Callback Functions PHP JSON PHP AND Exceptions PHP Connect database
-
MY SQL
SQL Introduction Syntax Select statement Select Distinct WHERE Clause Order By SQL AND Operator SQL OR Operator SQL NOT Operator SQL LIKE SQL IN SQL BETWEEN SQL INSERT INTO SQL NULL Values SQL UPDATE SQL DELETE SQL TOP, LIMIT, FETCH FIRST or ROWNUM Clause SQL MIN() and MAX() Functions SQL COUNT() Function SQL SUM() SQL AVG() SQL Aliases SQL JOIN SQL INNER JOIN SQL LEFT JOIN SQL RIGHT JOIN SQL FULL OUTER JOIN SQL Self Join SQL UNION SQL GROUP BY SQL HAVING SQL EXISTS SQL ANY and ALL SQL SELECT INTO SQL INSERT INTO SELECT SQL CASE SQL NULL Functions SQL Stored Procedures SQL Comments SQL Operators SQL CREATE DATABASE SQL DROP DATABASE SQL BACKUP DATABASE SQL CREATE TABLE SQL DROP TABLE SQL ALTER TABLE SQL Constraints SQL NOT NULL SQL UNIQUE Constraint SQL PRIMARY KEY SQL FOREIGN KEY SQL CHECK Constraint SQL CREATE INDEX SQL AUTO INCREMENT SQL Dates SQL Views SQL Injection SQL Hosting SQL Data Types
$.each() in jquery
The $.each()
function in jQuery is a versatile utility that allows you to iterate over arrays and objects. It executes a function for each element in the collection, making it useful for tasks like manipulating or accessing data within arrays or objects.
Syntax
For Arrays:
$.each(array, function(index, value) {
// Code to execute for each element
});
For Objects:
$.each(object, function(key, value) {
// Code to execute for each property
});
Parameters
- array/object: The array or object to iterate over.
- function(index/key, value): A callback function that is executed for each element or property.
- index/key: The index (for arrays) or key (for objects) of the current element or property.
- value: The value of the current element or property.
Return Value
$.each()
always returns the original collection, which allows for chaining.
Examples
Iterating Over an Array
var fruits = ['apple', 'banana', 'cherry'];
$.each(fruits, function(index, value) {
console.log(index + ': ' + value);
});
Output:
0: apple
1: banana
2: cherry
Iterating Over an Object
var person = {
name: 'John',
age: 30,
city: 'New York'
};
$.each(person, function(key, value) {
console.log(key + ': ' + value);
});
Output:
name: John
age: 30
city: New York
Key Points
- Order of Iteration: For arrays,
$.each()
iterates from index 0 to the end. For objects, it iterates over the properties in an arbitrary order. - Modification: You can modify the elements or properties directly within the callback function.
- Early Exit: To stop iteration early, you can return
false
from the callback function.
$.each(fruits, function(index, value) {
if (value === 'banana') {
return false; // Stops the iteration
}
console.log(index + ': ' + value);
});
Output:
0: apple
1: banana
The $.each()
function is a powerful tool in jQuery for handling collections of data and is particularly useful for working with dynamic content.
At Online Learner, we're on a mission to ignite a passion for learning and empower individuals to reach their full potential. Founded by a team of dedicated educators and industry experts, our platform is designed to provide accessible and engaging educational resources for learners of all ages and backgrounds.
Copyright 2023-2025 © All rights reserved.