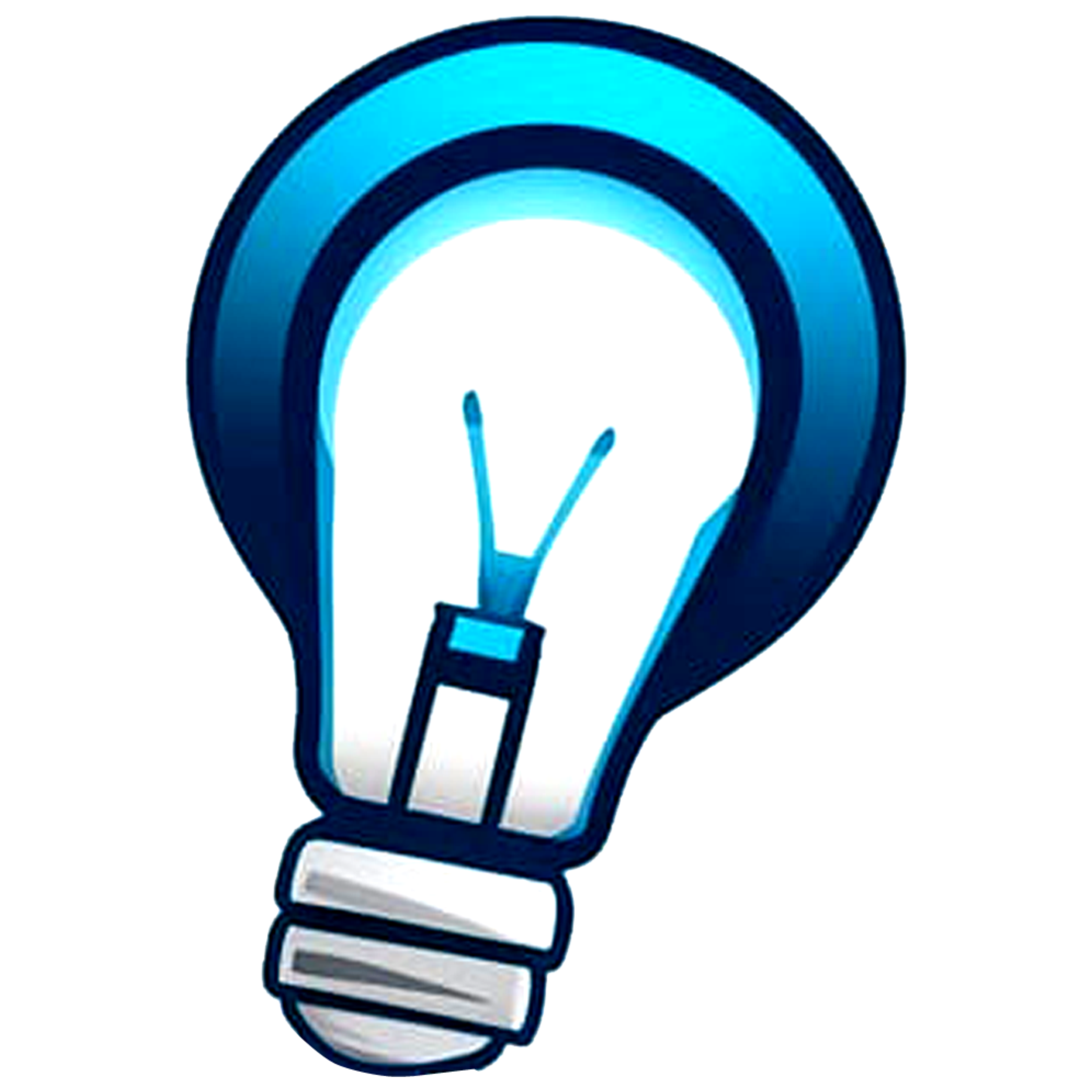
- Home
-
HTML
HTML Introduction HTML Tags HTML Elements HTML Attributes HTML Heading HTML Paragraph HTML Formatting HTML Quotations HTML Comments HTML Styles HTML Color HTML CSS HTML Images HTML Favicon HTML Links HTML DIV HTML Tables HTML Table Size HTML Table Head Table Padding & Spacing Table colspan rowspsn HTML Table Styling HTML Colgroup HTML List HTML Block & Inline HTML Classes HTML Id HTML Iframes HTML Head HTML Layout HTML Semantic Elements HTML Style Guide HTML Forms HTML Form Attribute HTML Form Element HTML input type HTML Computer code HTML Entity HTML Symbol HTML Emojis HTML Charset HTML Input Form Attribute HTML URL Encoding
-
CSS
CSS Introduction CSS Syntax CSS Selector How To Add CSS CSS Comments CSS Colors CSS Background color CSS background-image CSS Borders CSS Margins CSS Height, Width and Max-width CSS Box Model CSS Outline CSS Text CSS Fonts CSS Icon CSS Links CSS Tables CSS Display CSS Maximum Width CSS Position z-index Property
- JavaScript
-
JQuery
What is jQuery? Benefits of using jQuery Include jQuery Selectors. Methods. The $ symbol and shorthand. Selecting elements Getting and setting content Adding and removing elements Modifying CSS and classes Binding and Unbinding events Common events: click, hover, focus, blur, etc Event delegation Using .on() for dynamic content Showing and hiding elements Fading elements in and out Sliding elements up and down .animate() Understanding AJAX .ajax() .load(), .get(), .post() Handling responses and errors. Parent Chlid Siblings Filtering Elements Using find Selecting form elements Getting form values Setting form values Form validation Handling form submissions jQuery plugins Sliders plugins $.each() $.trim() $.extend() Data attributes Debugging jQuery code
-
Bootstrap 4
What is Bootstrap Benefits of using Setting up Container Row and Column Grid Classes Breakpoints Offsetting Columns Column Ordering Basic Typography Text Alignment Text colors Backgrounds Display Font Size Utilities Buttons Navs and Navbar Forms Cards Alerts Badges Progress Bars Margin Padding Sizing Flexbox Dropdowns Modals Tooltips Popovers Collapse Carousel Images Tables Jumbotron Media Object
- Git
-
PHP
PHP Introduction PHP Installation PHP Syntax PHP Comments PHP Variable PHP Echo PHP Data Types PHP Strings PHP Constant PHP Maths PHP Number PHP Operators PHP if else & if else if PHP Switch PHP Loops PHP Functions PHP Array PHP OOps PHP Class & Object PHP Constructor PHP Destructor PHP Access Modfiers PHP Inheritance PHP Final Keyword PHP Class Constant PHP Abstract Class PHP Superglobals PHP Regular Expression PHP Interfaces PHP Static Method PHP Static Properties PHP Namespace PHP Iterable PHP Form Introduction PHP Form Validation PHP Complete Form PHP Date and Time PHP Include Files PHP - Files & I/O File Upload PHP Cookies PHP SESSION PHP Filters PHP Callback Functions PHP JSON PHP AND Exceptions PHP Connect database
-
MY SQL
SQL Introduction Syntax Select statement Select Distinct WHERE Clause Order By SQL AND Operator SQL OR Operator SQL NOT Operator SQL LIKE SQL IN SQL BETWEEN SQL INSERT INTO SQL NULL Values SQL UPDATE SQL DELETE SQL TOP, LIMIT, FETCH FIRST or ROWNUM Clause SQL MIN() and MAX() Functions SQL COUNT() Function SQL SUM() SQL AVG() SQL Aliases SQL JOIN SQL INNER JOIN SQL LEFT JOIN SQL RIGHT JOIN SQL FULL OUTER JOIN SQL Self Join SQL UNION SQL GROUP BY SQL HAVING SQL EXISTS SQL ANY and ALL SQL SELECT INTO SQL INSERT INTO SELECT SQL CASE SQL NULL Functions SQL Stored Procedures SQL Comments SQL Operators SQL CREATE DATABASE SQL DROP DATABASE SQL BACKUP DATABASE SQL CREATE TABLE SQL DROP TABLE SQL ALTER TABLE SQL Constraints SQL NOT NULL SQL UNIQUE Constraint SQL PRIMARY KEY SQL FOREIGN KEY SQL CHECK Constraint SQL CREATE INDEX SQL AUTO INCREMENT SQL Dates SQL Views SQL Injection SQL Hosting SQL Data Types
Explain PHP JSON
PHP provides built-in functions to work with JSON (JavaScript Object Notation), a popular data interchange format. These functions are json_encode
and json_decode
.
Encoding JSON
json_encode
converts a PHP variable (such as an array or object) into a JSON string.
Example 1: Encoding an array to JSON
<?php
$data = array(
"name" => "John Doe",
"age" => 30,
"city" => "New York"
);
$json = json_encode($data);
echo $json; // Output: {"name":"John Doe","age":30,"city":"New York"}
?>
Example 2: Encoding a multi-dimensional array
<?php
$data = array(
"employees" => array(
array("name" => "John Doe", "age" => 30, "city" => "New York"),
array("name" => "Jane Doe", "age" => 25, "city" => "Los Angeles")
),
"company" => "Tech Solutions"
);
$json = json_encode($data, JSON_PRETTY_PRINT);
echo $json;
/*
Output:
{
"employees": [
{
"name": "John Doe",
"age": 30,
"city": "New York"
},
{
"name": "Jane Doe",
"age": 25,
"city": "Los Angeles"
}
],
"company": "Tech Solutions"
}
*/
?>
Decoding JSON
json_decode
converts a JSON string into a PHP variable.
Example 1: Decoding a JSON string to an associative array
<?php
$json = '{"name":"John Doe","age":30,"city":"New York"}';
$data = json_decode($json, true);
print_r($data);
/*
Output:
Array
(
[name] => John Doe
[age] => 30
[city] => New York
)
*/
?>
Example 2: Decoding a JSON string to an object
<?php
$json = '{"name":"John Doe","age":30,"city":"New York"}';
$data = json_decode($json);
echo $data->name; // Output: John Doe
echo $data->age; // Output: 30
echo $data->city; // Output: New York
?>
Example 3: Handling complex JSON structure
<?php
$json = '{
"employees": [
{"name": "John Doe", "age": 30, "city": "New York"},
{"name": "Jane Doe", "age": 25, "city": "Los Angeles"}
],
"company": "Tech Solutions"
}';
$data = json_decode($json, true);
print_r($data);
/*
Output:
Array
(
[employees] => Array
(
[0] => Array
(
[name] => John Doe
[age] => 30
[city] => New York
)
[1] => Array
(
[name] => Jane Doe
[age] => 25
[city] => Los Angeles
)
)
[company] => Tech Solutions
)
*/
?>
Handling JSON Errors
PHP provides json_last_error
and json_last_error_msg
functions to check for errors during JSON encoding or decoding.
Example: Checking for JSON errors
<?php
$json = '{"name":"John Doe", "age":30, "city":"New York"'; // Missing closing }
$data = json_decode($json);
if (json_last_error() != JSON_ERROR_NONE) {
echo "JSON Error: " . json_last_error_msg(); // Output: JSON Error: Syntax error
}
?>
These are the basic operations you can perform with JSON in PHP. Using json_encode
and json_decode
, you can easily convert between PHP data structures and JSON format for data interchange.
At Online Learner, we're on a mission to ignite a passion for learning and empower individuals to reach their full potential. Founded by a team of dedicated educators and industry experts, our platform is designed to provide accessible and engaging educational resources for learners of all ages and backgrounds.
Copyright 2023-2025 © All rights reserved.