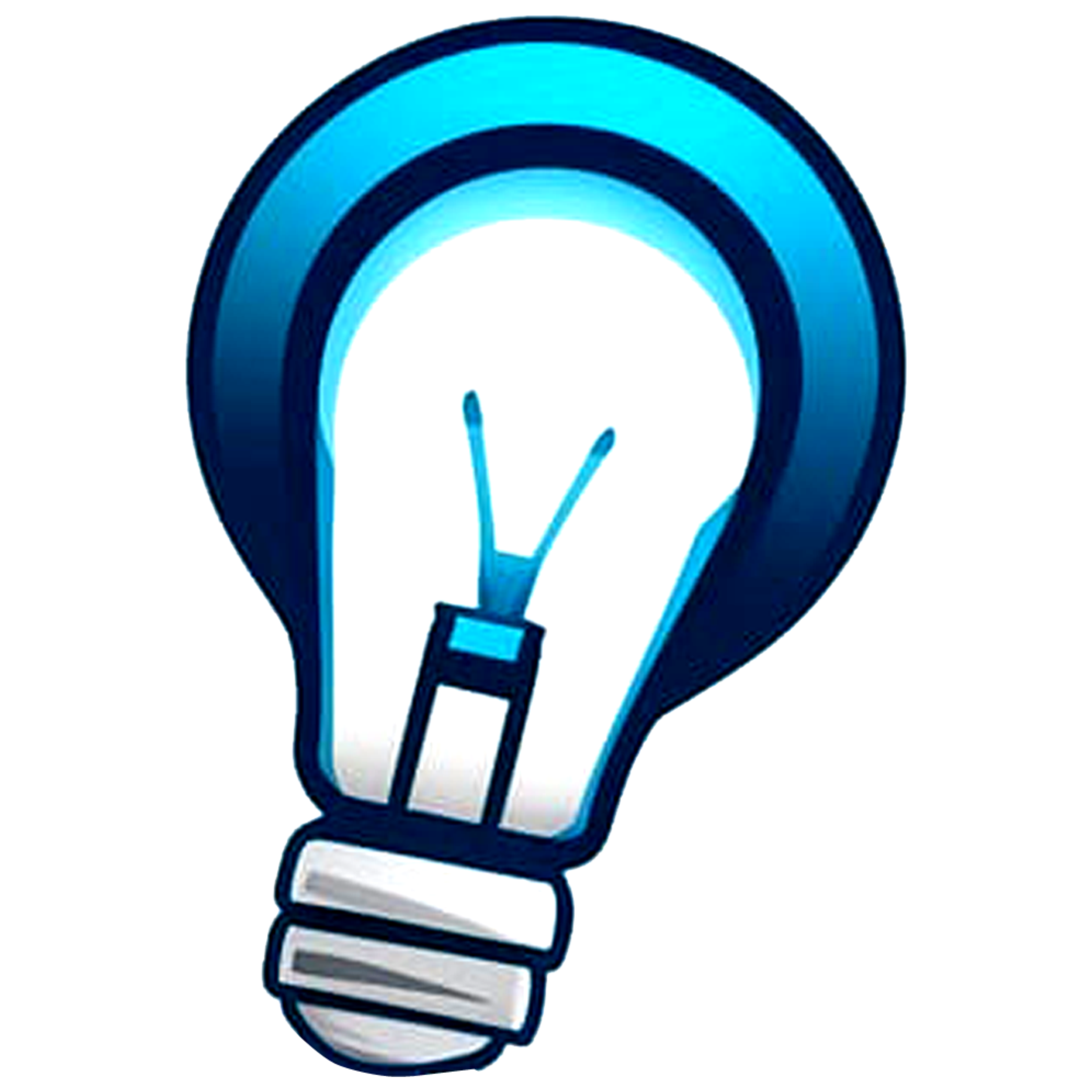
- Home
-
HTML
HTML Introduction HTML Tags HTML Elements HTML Attributes HTML Heading HTML Paragraph HTML Formatting HTML Quotations HTML Comments HTML Styles HTML Color HTML CSS HTML Images HTML Favicon HTML Links HTML DIV HTML Tables HTML Table Size HTML Table Head Table Padding & Spacing Table colspan rowspsn HTML Table Styling HTML Colgroup HTML List HTML Block & Inline HTML Classes HTML Id HTML Iframes HTML Head HTML Layout HTML Semantic Elements HTML Style Guide HTML Forms HTML Form Attribute HTML Form Element HTML input type HTML Computer code HTML Entity HTML Symbol HTML Emojis HTML Charset HTML Input Form Attribute HTML URL Encoding
-
CSS
CSS Introduction CSS Syntax CSS Selector How To Add CSS CSS Comments CSS Colors CSS Background color CSS background-image CSS Borders CSS Margins CSS Height, Width and Max-width CSS Box Model CSS Outline CSS Text CSS Fonts CSS Icon CSS Links CSS Tables CSS Display CSS Maximum Width CSS Position z-index Property
- JavaScript
-
JQuery
What is jQuery? Benefits of using jQuery Include jQuery Selectors. Methods. The $ symbol and shorthand. Selecting elements Getting and setting content Adding and removing elements Modifying CSS and classes Binding and Unbinding events Common events: click, hover, focus, blur, etc Event delegation Using .on() for dynamic content Showing and hiding elements Fading elements in and out Sliding elements up and down .animate() Understanding AJAX .ajax() .load(), .get(), .post() Handling responses and errors. Parent Chlid Siblings Filtering Elements Using find Selecting form elements Getting form values Setting form values Form validation Handling form submissions jQuery plugins Sliders plugins $.each() $.trim() $.extend() Data attributes Debugging jQuery code
-
Bootstrap 4
What is Bootstrap Benefits of using Setting up Container Row and Column Grid Classes Breakpoints Offsetting Columns Column Ordering Basic Typography Text Alignment Text colors Backgrounds Display Font Size Utilities Buttons Navs and Navbar Forms Cards Alerts Badges Progress Bars Margin Padding Sizing Flexbox Dropdowns Modals Tooltips Popovers Collapse Carousel Images Tables Jumbotron Media Object
- Git
-
PHP
PHP Introduction PHP Installation PHP Syntax PHP Comments PHP Variable PHP Echo PHP Data Types PHP Strings PHP Constant PHP Maths PHP Number PHP Operators PHP if else & if else if PHP Switch PHP Loops PHP Functions PHP Array PHP OOps PHP Class & Object PHP Constructor PHP Destructor PHP Access Modfiers PHP Inheritance PHP Final Keyword PHP Class Constant PHP Abstract Class PHP Superglobals PHP Regular Expression PHP Interfaces PHP Static Method PHP Static Properties PHP Namespace PHP Iterable PHP Form Introduction PHP Form Validation PHP Complete Form PHP Date and Time PHP Include Files PHP - Files & I/O File Upload PHP Cookies PHP SESSION PHP Filters PHP Callback Functions PHP JSON PHP AND Exceptions PHP Connect database
-
MY SQL
SQL Introduction Syntax Select statement Select Distinct WHERE Clause Order By SQL AND Operator SQL OR Operator SQL NOT Operator SQL LIKE SQL IN SQL BETWEEN SQL INSERT INTO SQL NULL Values SQL UPDATE SQL DELETE SQL TOP, LIMIT, FETCH FIRST or ROWNUM Clause SQL MIN() and MAX() Functions SQL COUNT() Function SQL SUM() SQL AVG() SQL Aliases SQL JOIN SQL INNER JOIN SQL LEFT JOIN SQL RIGHT JOIN SQL FULL OUTER JOIN SQL Self Join SQL UNION SQL GROUP BY SQL HAVING SQL EXISTS SQL ANY and ALL SQL SELECT INTO SQL INSERT INTO SELECT SQL CASE SQL NULL Functions SQL Stored Procedures SQL Comments SQL Operators SQL CREATE DATABASE SQL DROP DATABASE SQL BACKUP DATABASE SQL CREATE TABLE SQL DROP TABLE SQL ALTER TABLE SQL Constraints SQL NOT NULL SQL UNIQUE Constraint SQL PRIMARY KEY SQL FOREIGN KEY SQL CHECK Constraint SQL CREATE INDEX SQL AUTO INCREMENT SQL Dates SQL Views SQL Injection SQL Hosting SQL Data Types
Explain JavaScript Operators
Sure! JavaScript operators are symbols used to perform operations on operands (values and variables). They can be categorized into several types:
1. Arithmetic Operators
These operators perform basic arithmetic operations.
-
Addition (+): Adds two numbers.
let a = 5; let b = 3; console.log(a + b); // Output: 8
-
Subtraction (-): Subtracts the second number from the first.
console.log(a - b); // Output: 2
-
Multiplication (*): Multiplies two numbers.
console.log(a * b); // Output: 15
-
Division (/): Divides the first number by the second.
console.log(a / b); // Output: 1.6666666666666667
-
Modulus (%): Returns the remainder of the division.
console.log(a % b); // Output: 2
-
Exponentiation ()**: Raises the first number to the power of the second.
console.log(a ** b); // Output: 125
2. Assignment Operators
These operators assign values to variables.
-
Assignment (=): Assigns the value on the right to the variable on the left.
let c = 10;
-
Addition Assignment (+=): Adds the right operand to the left operand and assigns the result to the left operand.
c += 5; // Equivalent to c = c + 5 console.log(c); // Output: 15
-
Subtraction Assignment (-=): Subtracts the right operand from the left operand and assigns the result to the left operand.
c -= 3; // Equivalent to c = c - 3 console.log(c); // Output: 12
-
Multiplication Assignment (*=): Multiplies the left operand by the right operand and assigns the result to the left operand.
c *= 2; // Equivalent to c = c * 2 console.log(c); // Output: 24
-
Division Assignment (/=): Divides the left operand by the right operand and assigns the result to the left operand.
c /= 4; // Equivalent to c = c / 4 console.log(c); // Output: 6
3. Comparison Operators
These operators compare two values and return a boolean value.
-
Equal (==): Checks if two values are equal.
console.log(a == 5); // Output: true
-
Not Equal (!=): Checks if two values are not equal.
console.log(a != 5); // Output: false
-
Strict Equal (===): Checks if two values are equal and of the same type.
console.log(a === 5); // Output: true
-
Strict Not Equal (!==): Checks if two values are not equal or not of the same type.
console.log(a !== "5"); // Output: true
-
Greater Than (>): Checks if the left value is greater than the right value.
console.log(a > b); // Output: true
-
Less Than (<): Checks if the left value is less than the right value.
console.log(a < b); // Output: false
-
Greater Than or Equal (>=): Checks if the left value is greater than or equal to the right value.
console.log(a >= b); // Output: true
-
Less Than or Equal (<=): Checks if the left value is less than or equal to the right value.
console.log(a <= b); // Output: false
4. Logical Operators
These operators perform logical operations.
-
AND (&&): Returns true if both operands are true.
console.log(true && false); // Output: false
-
OR (||): Returns true if at least one of the operands is true.
console.log(true || false); // Output: true
-
NOT (!): Inverts the boolean value.
console.log(!true); // Output: false
5. Bitwise Operators
These operators perform bit-level operations.
-
AND (&): Performs a bitwise AND.
console.log(a & b); // Output: 1 (binary: 0101 & 0011 = 0001)
-
OR (|): Performs a bitwise OR.
console.log(a | b); // Output: 7 (binary: 0101 | 0011 = 0111)
-
XOR (^): Performs a bitwise XOR.
console.log(a ^ b); // Output: 6 (binary: 0101 ^ 0011 = 0110)
-
NOT (~): Performs a bitwise NOT.
console.log(~a); // Output: -6 (binary: ~0101 = 1010)
-
Left Shift (<<): Shifts bits to the left.
console.log(a << 1); // Output: 10 (binary: 0101 << 1 = 1010)
-
Right Shift (>>): Shifts bits to the right.
console.log(a >> 1); // Output: 2 (binary: 0101 >> 1 = 0010)
6. Ternary Operator
The ternary operator is a shorthand for an if-else statement.
- Conditional (?:): Returns a value based on a condition.
let isAdult = (a > 18) ? "Yes" : "No"; console.log(isAdult); // Output: No
7. Type Operators
These operators determine the type of a variable.
-
typeof: Returns the type of a variable.
console.log(typeof a); // Output: number
-
instanceof: Checks if an object is an instance of a specific class.
let date = new Date(); console.log(date instanceof Date); // Output: true
These are the basic JavaScript operators with examples to illustrate their usage.
At Online Learner, we're on a mission to ignite a passion for learning and empower individuals to reach their full potential. Founded by a team of dedicated educators and industry experts, our platform is designed to provide accessible and engaging educational resources for learners of all ages and backgrounds.
Copyright 2023-2025 © All rights reserved.