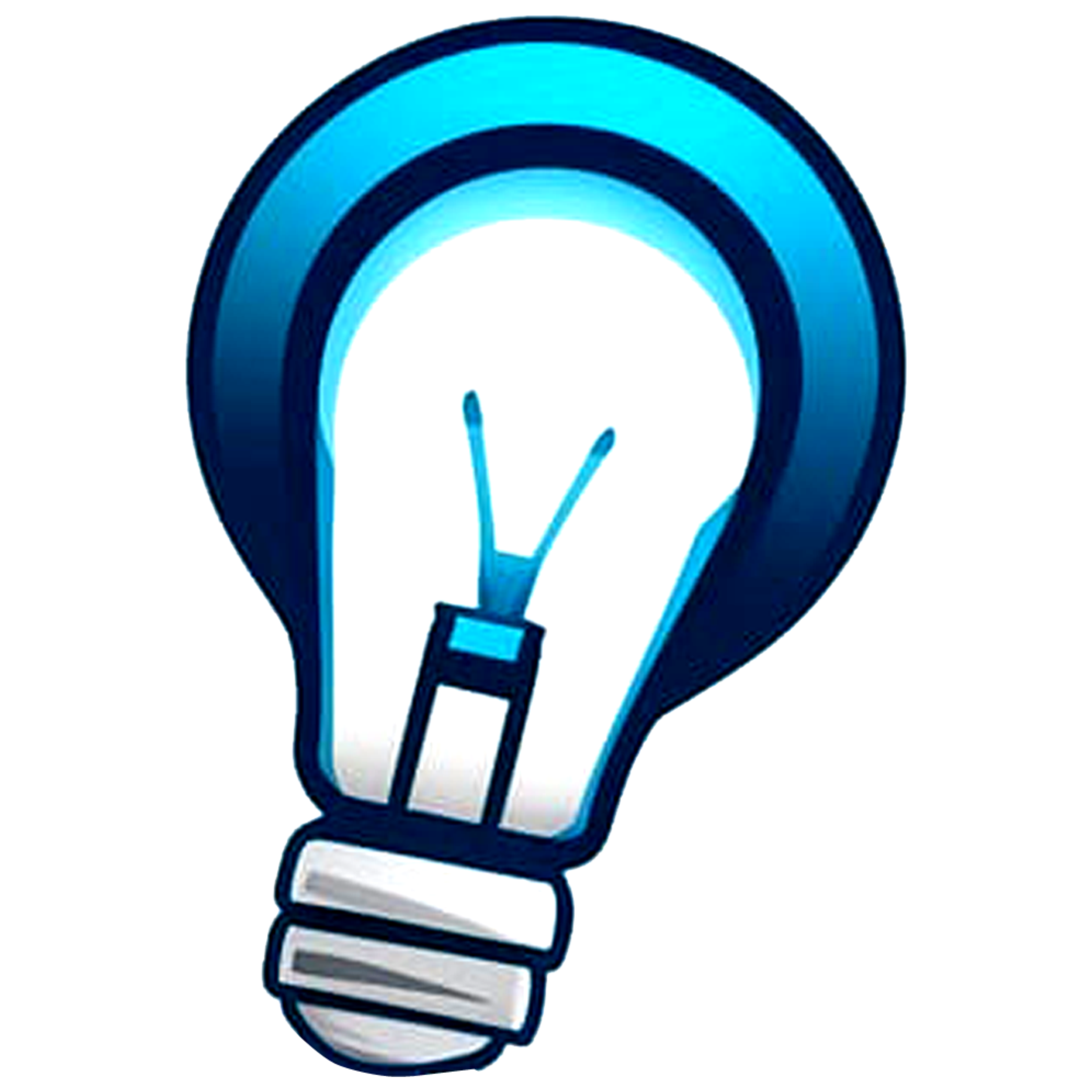
- Home
-
HTML
HTML Introduction HTML Tags HTML Elements HTML Attributes HTML Heading HTML Paragraph HTML Formatting HTML Quotations HTML Comments HTML Styles HTML Color HTML CSS HTML Images HTML Favicon HTML Links HTML DIV HTML Tables HTML Table Size HTML Table Head Table Padding & Spacing Table colspan rowspsn HTML Table Styling HTML Colgroup HTML List HTML Block & Inline HTML Classes HTML Id HTML Iframes HTML Head HTML Layout HTML Semantic Elements HTML Style Guide HTML Forms HTML Form Attribute HTML Form Element HTML input type HTML Computer code HTML Entity HTML Symbol HTML Emojis HTML Charset HTML Input Form Attribute HTML URL Encoding
-
CSS
CSS Introduction CSS Syntax CSS Selector How To Add CSS CSS Comments CSS Colors CSS Background color CSS background-image CSS Borders CSS Margins CSS Height, Width and Max-width CSS Box Model CSS Outline CSS Text CSS Fonts CSS Icon CSS Links CSS Tables CSS Display CSS Maximum Width CSS Position z-index Property
- JavaScript
-
JQuery
What is jQuery? Benefits of using jQuery Include jQuery Selectors. Methods. The $ symbol and shorthand. Selecting elements Getting and setting content Adding and removing elements Modifying CSS and classes Binding and Unbinding events Common events: click, hover, focus, blur, etc Event delegation Using .on() for dynamic content Showing and hiding elements Fading elements in and out Sliding elements up and down .animate() Understanding AJAX .ajax() .load(), .get(), .post() Handling responses and errors. Parent Chlid Siblings Filtering Elements Using find Selecting form elements Getting form values Setting form values Form validation Handling form submissions jQuery plugins Sliders plugins $.each() $.trim() $.extend() Data attributes Debugging jQuery code
-
Bootstrap 4
What is Bootstrap Benefits of using Setting up Container Row and Column Grid Classes Breakpoints Offsetting Columns Column Ordering Basic Typography Text Alignment Text colors Backgrounds Display Font Size Utilities Buttons Navs and Navbar Forms Cards Alerts Badges Progress Bars Margin Padding Sizing Flexbox Dropdowns Modals Tooltips Popovers Collapse Carousel Images Tables Jumbotron Media Object
- Git
-
PHP
PHP Introduction PHP Installation PHP Syntax PHP Comments PHP Variable PHP Echo PHP Data Types PHP Strings PHP Constant PHP Maths PHP Number PHP Operators PHP if else & if else if PHP Switch PHP Loops PHP Functions PHP Array PHP OOps PHP Class & Object PHP Constructor PHP Destructor PHP Access Modfiers PHP Inheritance PHP Final Keyword PHP Class Constant PHP Abstract Class PHP Superglobals PHP Regular Expression PHP Interfaces PHP Static Method PHP Static Properties PHP Namespace PHP Iterable PHP Form Introduction PHP Form Validation PHP Complete Form PHP Date and Time PHP Include Files PHP - Files & I/O File Upload PHP Cookies PHP SESSION PHP Filters PHP Callback Functions PHP JSON PHP AND Exceptions PHP Connect database
-
MY SQL
SQL Introduction Syntax Select statement Select Distinct WHERE Clause Order By SQL AND Operator SQL OR Operator SQL NOT Operator SQL LIKE SQL IN SQL BETWEEN SQL INSERT INTO SQL NULL Values SQL UPDATE SQL DELETE SQL TOP, LIMIT, FETCH FIRST or ROWNUM Clause SQL MIN() and MAX() Functions SQL COUNT() Function SQL SUM() SQL AVG() SQL Aliases SQL JOIN SQL INNER JOIN SQL LEFT JOIN SQL RIGHT JOIN SQL FULL OUTER JOIN SQL Self Join SQL UNION SQL GROUP BY SQL HAVING SQL EXISTS SQL ANY and ALL SQL SELECT INTO SQL INSERT INTO SELECT SQL CASE SQL NULL Functions SQL Stored Procedures SQL Comments SQL Operators SQL CREATE DATABASE SQL DROP DATABASE SQL BACKUP DATABASE SQL CREATE TABLE SQL DROP TABLE SQL ALTER TABLE SQL Constraints SQL NOT NULL SQL UNIQUE Constraint SQL PRIMARY KEY SQL FOREIGN KEY SQL CHECK Constraint SQL CREATE INDEX SQL AUTO INCREMENT SQL Dates SQL Views SQL Injection SQL Hosting SQL Data Types
Explain Javascript Switch Statement
The switch
statement in JavaScript is used to execute one block of code among many options. It is an alternative to using multiple if...else if
statements, making the code more readable and easier to manage when dealing with multiple possible values for a single variable.
Here's the basic syntax of a switch
statement:
switch (expression) {
case value1:
// Code to be executed if expression === value1
break;
case value2:
// Code to be executed if expression === value2
break;
// You can have as many cases as needed
default:
// Code to be executed if expression doesn't match any case
}
Example 1: Basic Switch Statement
Let's use a switch statement to display the name of the day based on a number (0 for Sunday, 1 for Monday, etc.).
let day = 3;
let dayName;
switch (day) {
case 0:
dayName = "Sunday";
break;
case 1:
dayName = "Monday";
break;
case 2:
dayName = "Tuesday";
break;
case 3:
dayName = "Wednesday";
break;
case 4:
dayName = "Thursday";
break;
case 5:
dayName = "Friday";
break;
case 6:
dayName = "Saturday";
break;
default:
dayName = "Invalid day";
}
console.log(dayName); // Output: Wednesday
Example 2: Using Default Case
If the value of the expression doesn't match any of the specified cases, the code inside the default
case will be executed.
let fruit = "Mango";
let color;
switch (fruit) {
case "Banana":
color = "Yellow";
break;
case "Apple":
color = "Red";
break;
case "Grapes":
color = "Purple";
break;
default:
color = "Unknown";
}
console.log(color); // Output: Unknown
Example 3: Grouping Cases
You can group cases to execute the same code for multiple values.
let animal = "Dog";
let sound;
switch (animal) {
case "Cow":
case "Goat":
case "Sheep":
sound = "Baa";
break;
case "Dog":
case "Cat":
sound = "Woof or Meow";
break;
default:
sound = "Unknown sound";
}
console.log(sound); // Output: Woof or Meow
Example 4: Fall-Through
If you omit the break
statement, the code will continue to execute the following cases until a break
is encountered. This is known as "fall-through."
let grade = 'B';
let message;
switch (grade) {
case 'A':
message = "Excellent";
break;
case 'B':
case 'C':
message = "Well done";
break;
case 'D':
message = "You passed";
break;
case 'F':
message = "Better try again";
break;
default:
message = "Unknown grade";
}
console.log(message); // Output: Well done
In this example, both 'B' and 'C' will result in the message "Well done" because the case 'C':
does not have a break
and thus "falls through" to the case 'B':
block.
Using the switch
statement can make your code cleaner and easier to understand when dealing with multiple possible values for a single variable.
At Online Learner, we're on a mission to ignite a passion for learning and empower individuals to reach their full potential. Founded by a team of dedicated educators and industry experts, our platform is designed to provide accessible and engaging educational resources for learners of all ages and backgrounds.
Copyright 2023-2024 © All rights reserved.