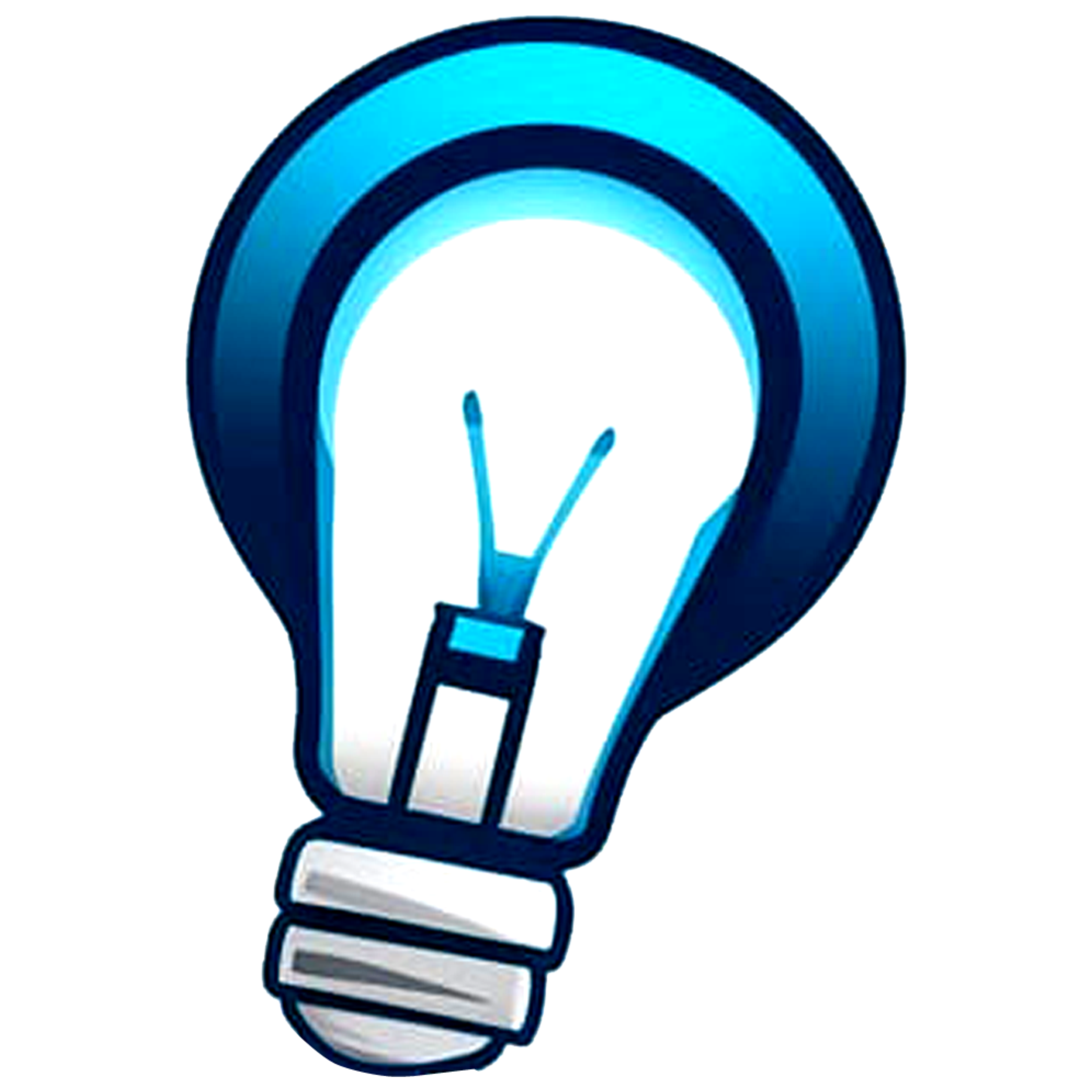
- Home
-
HTML
HTML Introduction HTML Tags HTML Elements HTML Attributes HTML Heading HTML Paragraph HTML Formatting HTML Quotations HTML Comments HTML Styles HTML Color HTML CSS HTML Images HTML Favicon HTML Links HTML DIV HTML Tables HTML Table Size HTML Table Head Table Padding & Spacing Table colspan rowspsn HTML Table Styling HTML Colgroup HTML List HTML Block & Inline HTML Classes HTML Id HTML Iframes HTML Head HTML Layout HTML Semantic Elements HTML Style Guide HTML Forms HTML Form Attribute HTML Form Element HTML input type HTML Computer code HTML Entity HTML Symbol HTML Emojis HTML Charset HTML Input Form Attribute HTML URL Encoding
-
CSS
CSS Introduction CSS Syntax CSS Selector How To Add CSS CSS Comments CSS Colors CSS Background color CSS background-image CSS Borders CSS Margins CSS Height, Width and Max-width CSS Box Model CSS Outline CSS Text CSS Fonts CSS Icon CSS Links CSS Tables CSS Display CSS Maximum Width CSS Position z-index Property
- JavaScript
-
JQuery
What is jQuery? Benefits of using jQuery Include jQuery Selectors. Methods. The $ symbol and shorthand. Selecting elements Getting and setting content Adding and removing elements Modifying CSS and classes Binding and Unbinding events Common events: click, hover, focus, blur, etc Event delegation Using .on() for dynamic content Showing and hiding elements Fading elements in and out Sliding elements up and down .animate() Understanding AJAX .ajax() .load(), .get(), .post() Handling responses and errors. Parent Chlid Siblings Filtering Elements Using find Selecting form elements Getting form values Setting form values Form validation Handling form submissions jQuery plugins Sliders plugins $.each() $.trim() $.extend() Data attributes Debugging jQuery code
-
Bootstrap 4
What is Bootstrap Benefits of using Setting up Container Row and Column Grid Classes Breakpoints Offsetting Columns Column Ordering Basic Typography Text Alignment Text colors Backgrounds Display Font Size Utilities Buttons Navs and Navbar Forms Cards Alerts Badges Progress Bars Margin Padding Sizing Flexbox Dropdowns Modals Tooltips Popovers Collapse Carousel Images Tables Jumbotron Media Object
- Git
-
PHP
PHP Introduction PHP Installation PHP Syntax PHP Comments PHP Variable PHP Echo PHP Data Types PHP Strings PHP Constant PHP Maths PHP Number PHP Operators PHP if else & if else if PHP Switch PHP Loops PHP Functions PHP Array PHP OOps PHP Class & Object PHP Constructor PHP Destructor PHP Access Modfiers PHP Inheritance PHP Final Keyword PHP Class Constant PHP Abstract Class PHP Superglobals PHP Regular Expression PHP Interfaces PHP Static Method PHP Static Properties PHP Namespace PHP Iterable PHP Form Introduction PHP Form Validation PHP Complete Form PHP Date and Time PHP Include Files PHP - Files & I/O File Upload PHP Cookies PHP SESSION PHP Filters PHP Callback Functions PHP JSON PHP AND Exceptions PHP Connect database
-
MY SQL
SQL Introduction Syntax Select statement Select Distinct WHERE Clause Order By SQL AND Operator SQL OR Operator SQL NOT Operator SQL LIKE SQL IN SQL BETWEEN SQL INSERT INTO SQL NULL Values SQL UPDATE SQL DELETE SQL TOP, LIMIT, FETCH FIRST or ROWNUM Clause SQL MIN() and MAX() Functions SQL COUNT() Function SQL SUM() SQL AVG() SQL Aliases SQL JOIN SQL INNER JOIN SQL LEFT JOIN SQL RIGHT JOIN SQL FULL OUTER JOIN SQL Self Join SQL UNION SQL GROUP BY SQL HAVING SQL EXISTS SQL ANY and ALL SQL SELECT INTO SQL INSERT INTO SELECT SQL CASE SQL NULL Functions SQL Stored Procedures SQL Comments SQL Operators SQL CREATE DATABASE SQL DROP DATABASE SQL BACKUP DATABASE SQL CREATE TABLE SQL DROP TABLE SQL ALTER TABLE SQL Constraints SQL NOT NULL SQL UNIQUE Constraint SQL PRIMARY KEY SQL FOREIGN KEY SQL CHECK Constraint SQL CREATE INDEX SQL AUTO INCREMENT SQL Dates SQL Views SQL Injection SQL Hosting SQL Data Types
Form validation using JQuery
Form validation using different parameters with jQuery involves checking user inputs in forms to ensure they meet certain criteria before the form is submitted. This process helps to improve the quality of data submitted and enhance user experience. Here's an explanation of how you can implement form validation with various parameters using jQuery:
Steps to Implement Form Validation with jQuery
-
Include jQuery Library: Make sure you include the jQuery library in your project. You can add it via a CDN or download it locally.
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
-
Create the HTML Form: Design your HTML form with the necessary input fields and a submit button.
<form id="myForm"> <label for="username">Username:</label> <input type="text" id="username" name="username"> <br> <label for="email">Email:</label> <input type="email" id="email" name="email"> <br> <label for="password">Password:</label> <input type="password" id="password" name="password"> <br> <input type="submit" value="Submit"> </form> <div id="errorMessages"></div>
-
Write the jQuery Validation Code: Use jQuery to validate the form inputs based on different parameters like required fields, email format, minimum length, etc.
$(document).ready(function() { $('#myForm').on('submit', function(event) { event.preventDefault(); // Prevent the form from submitting by default // Clear previous error messages $('#errorMessages').empty(); // Define validation rules var isValid = true; var username = $('#username').val(); var email = $('#email').val(); var password = $('#password').val(); var errorMessages = ''; // Username validation if (username.length < 5) { isValid = false; errorMessages += '<p>Username must be at least 5 characters long.</p>'; } // Email validation var emailPattern = /^[^\s@]+@[^\s@]+\.[^\s@]+$/; if (!emailPattern.test(email)) { isValid = false; errorMessages += '<p>Please enter a valid email address.</p>'; } // Password validation if (password.length < 8) { isValid = false; errorMessages += '<p>Password must be at least 8 characters long.</p>'; } // Display error messages or submit the form if (!isValid) { $('#errorMessages').html(errorMessages); } else { // Form is valid, you can submit it or perform other actions alert('Form is valid and ready to be submitted!'); // this.submit(); // Uncomment this line to submit the form } }); });
Explanation of the Code:
-
Include jQuery Library: Ensure jQuery is included to use its functionalities.
-
Create the HTML Form: Design the form with appropriate fields and a submit button.
-
jQuery Validation Code:
- Use
$(document).ready()
to ensure the DOM is fully loaded before running the script. - Attach a
submit
event handler to the form to prevent its default submission and perform validation. - Clear any previous error messages.
- Define the validation rules for each input field (e.g., username length, email format, password length).
- Use regular expressions to validate the email format.
- Display error messages if validation fails or submit the form if it passes.
- Use
This example demonstrates basic form validation using jQuery with different parameters. You can customize the validation rules and error messages based on your specific requirements.
At Online Learner, we're on a mission to ignite a passion for learning and empower individuals to reach their full potential. Founded by a team of dedicated educators and industry experts, our platform is designed to provide accessible and engaging educational resources for learners of all ages and backgrounds.
Copyright 2023-2025 © All rights reserved.