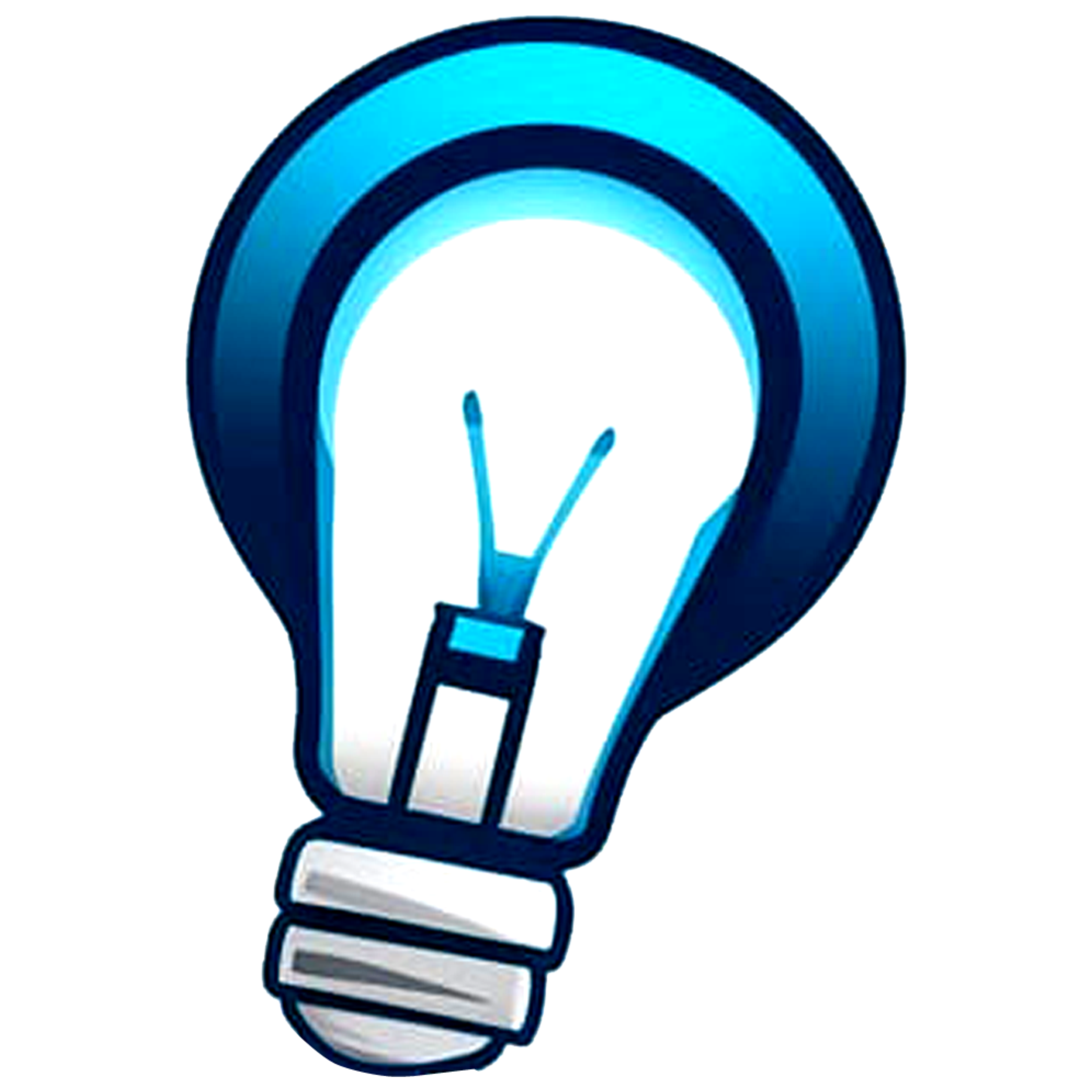
- Home
-
HTML
HTML Introduction HTML Tags HTML Elements HTML Attributes HTML Heading HTML Paragraph HTML Formatting HTML Quotations HTML Comments HTML Styles HTML Color HTML CSS HTML Images HTML Favicon HTML Links HTML DIV HTML Tables HTML Table Size HTML Table Head Table Padding & Spacing Table colspan rowspsn HTML Table Styling HTML Colgroup HTML List HTML Block & Inline HTML Classes HTML Id HTML Iframes HTML Head HTML Layout HTML Semantic Elements HTML Style Guide HTML Forms HTML Form Attribute HTML Form Element HTML input type HTML Computer code HTML Entity HTML Symbol HTML Emojis HTML Charset HTML Input Form Attribute HTML URL Encoding
-
CSS
CSS Introduction CSS Syntax CSS Selector How To Add CSS CSS Comments CSS Colors CSS Background color CSS background-image CSS Borders CSS Margins CSS Height, Width and Max-width CSS Box Model CSS Outline CSS Text CSS Fonts CSS Icon CSS Links CSS Tables CSS Display CSS Maximum Width CSS Position z-index Property
- JavaScript
-
JQuery
What is jQuery? Benefits of using jQuery Include jQuery Selectors. Methods. The $ symbol and shorthand. Selecting elements Getting and setting content Adding and removing elements Modifying CSS and classes Binding and Unbinding events Common events: click, hover, focus, blur, etc Event delegation Using .on() for dynamic content Showing and hiding elements Fading elements in and out Sliding elements up and down .animate() Understanding AJAX .ajax() .load(), .get(), .post() Handling responses and errors. Parent Chlid Siblings Filtering Elements Using find Selecting form elements Getting form values Setting form values Form validation Handling form submissions jQuery plugins Sliders plugins $.each() $.trim() $.extend() Data attributes Debugging jQuery code
-
Bootstrap 4
What is Bootstrap Benefits of using Setting up Container Row and Column Grid Classes Breakpoints Offsetting Columns Column Ordering Basic Typography Text Alignment Text colors Backgrounds Display Font Size Utilities Buttons Navs and Navbar Forms Cards Alerts Badges Progress Bars Margin Padding Sizing Flexbox Dropdowns Modals Tooltips Popovers Collapse Carousel Images Tables Jumbotron Media Object
- Git
-
PHP
PHP Introduction PHP Installation PHP Syntax PHP Comments PHP Variable PHP Echo PHP Data Types PHP Strings PHP Constant PHP Maths PHP Number PHP Operators PHP if else & if else if PHP Switch PHP Loops PHP Functions PHP Array PHP OOps PHP Class & Object PHP Constructor PHP Destructor PHP Access Modfiers PHP Inheritance PHP Final Keyword PHP Class Constant PHP Abstract Class PHP Superglobals PHP Regular Expression PHP Interfaces PHP Static Method PHP Static Properties PHP Namespace PHP Iterable PHP Form Introduction PHP Form Validation PHP Complete Form PHP Date and Time PHP Include Files PHP - Files & I/O File Upload PHP Cookies PHP SESSION PHP Filters PHP Callback Functions PHP JSON PHP AND Exceptions PHP Connect database
-
MY SQL
SQL Introduction Syntax Select statement Select Distinct WHERE Clause Order By SQL AND Operator SQL OR Operator SQL NOT Operator SQL LIKE SQL IN SQL BETWEEN SQL INSERT INTO SQL NULL Values SQL UPDATE SQL DELETE SQL TOP, LIMIT, FETCH FIRST or ROWNUM Clause SQL MIN() and MAX() Functions SQL COUNT() Function SQL SUM() SQL AVG() SQL Aliases SQL JOIN SQL INNER JOIN SQL LEFT JOIN SQL RIGHT JOIN SQL FULL OUTER JOIN SQL Self Join SQL UNION SQL GROUP BY SQL HAVING SQL EXISTS SQL ANY and ALL SQL SELECT INTO SQL INSERT INTO SELECT SQL CASE SQL NULL Functions SQL Stored Procedures SQL Comments SQL Operators SQL CREATE DATABASE SQL DROP DATABASE SQL BACKUP DATABASE SQL CREATE TABLE SQL DROP TABLE SQL ALTER TABLE SQL Constraints SQL NOT NULL SQL UNIQUE Constraint SQL PRIMARY KEY SQL FOREIGN KEY SQL CHECK Constraint SQL CREATE INDEX SQL AUTO INCREMENT SQL Dates SQL Views SQL Injection SQL Hosting SQL Data Types
Making AJAX requests with .ajax().
Making AJAX requests with .ajax()
in jQuery allows you to perform asynchronous HTTP requests. This method is versatile and can handle a wide range of request types, including GET, POST, PUT, DELETE, etc. Here's a basic overview of how to use .ajax()
:
Syntax
$.ajax({
url: 'your-url-here', // The URL to send the request to
type: 'GET', // The type of request (GET, POST, etc.)
data: { key1: 'value1' }, // Data to be sent to the server (optional)
dataType: 'json', // The type of data expected back from the server (optional)
success: function(response) {
// Code to run if the request succeeds
console.log(response);
},
error: function(jqXHR, textStatus, errorThrown) {
// Code to run if the request fails
console.error('Error: ' + textStatus, errorThrown);
}
});
Parameters
- url: The URL to which the request is sent.
- type: The type of request to make (
GET
,POST
,PUT
,DELETE
, etc.). The default isGET
. - data: Data to be sent to the server. This can be an object or a query string.
- dataType: The type of data expected back from the server (e.g.,
json
,xml
,html
,script
,text
). This is optional. - success: A callback function to be executed if the request is successful. The response from the server is passed as an argument to this function.
- error: A callback function to be executed if the request fails. It receives three arguments:
jqXHR
,textStatus
, anderrorThrown
.
Example: GET Request
$.ajax({
url: 'https://api.example.com/data',
type: 'GET',
dataType: 'json',
success: function(response) {
console.log('Data received:', response);
},
error: function(jqXHR, textStatus, errorThrown) {
console.error('Error fetching data:', textStatus, errorThrown);
}
});
Example: POST Request
$.ajax({
url: 'https://api.example.com/submit',
type: 'POST',
data: { name: 'John', age: 30 },
dataType: 'json',
success: function(response) {
console.log('Data submitted successfully:', response);
},
error: function(jqXHR, textStatus, errorThrown) {
console.error('Error submitting data:', textStatus, errorThrown);
}
});
Handling JSON Response
When the expected response is in JSON format, you can directly work with the JSON object in the success callback:
$.ajax({
url: 'https://api.example.com/user',
type: 'GET',
dataType: 'json',
success: function(user) {
console.log('User data:', user);
},
error: function(jqXHR, textStatus, errorThrown) {
console.error('Error fetching user data:', textStatus, errorThrown);
}
});
Error Handling
The error callback provides useful information about what went wrong. For instance, you can check the status code and the error message:
$.ajax({
url: 'https://api.example.com/data',
type: 'GET',
dataType: 'json',
success: function(response) {
console.log('Data received:', response);
},
error: function(jqXHR, textStatus, errorThrown) {
console.error('Error fetching data: ' + textStatus);
console.error('Error details:', errorThrown);
}
});
Using .ajax()
, you can effectively manage various types of HTTP requests, handle responses, and manage errors, making it a powerful tool for web development.
At Online Learner, we're on a mission to ignite a passion for learning and empower individuals to reach their full potential. Founded by a team of dedicated educators and industry experts, our platform is designed to provide accessible and engaging educational resources for learners of all ages and backgrounds.
Copyright 2023-2024 © All rights reserved.